Unit Testing in Software Testing: Types, Tools & Best Practices
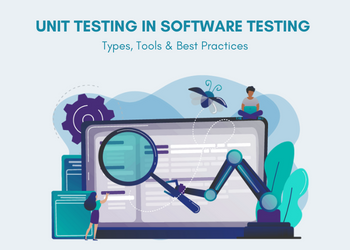
What is unit testing?
Unit Testing is a type of software testing in which individual software units or components are tested. The goal is to ensure that each unit of software code performs as expected. Developers perform unit test during an application’s development (coding phase). Unit tests isolate and verify the correctness of a section of code. A unit is a single function, method, procedure, module, or object.
Unit testing is the first level of testing performed before integration testing in SDLC, STLC, and V models. Unit test is a type of White Box testing that the developer typically performs. In practice, however, due to time constraints or developers’ reluctance to test, QA engineers also perform unit test.
Why is Unit Testing Necessary?
Unit testing is important because software developers often try to save time by performing minimal unit test; however, this is a myth because insufficient unit test leads to high-cost defect fixing during System Testing, Integration Testing, and even Beta Testing after the application is built. Proper unit testi during early development saves time and money in the long run.
The following are the primary reasons for performing unit test in software engineering:
- Unit tests aid in the early detection of bugs and the reduction of development costs.
- It allows developers to understand the testing code base and make changes quickly.
- Unit tests that are well-written serve as project documentation.
- Unit tests aid in code reuse. Transfer your code and tests to your new project. Change the code until the tests pass again.
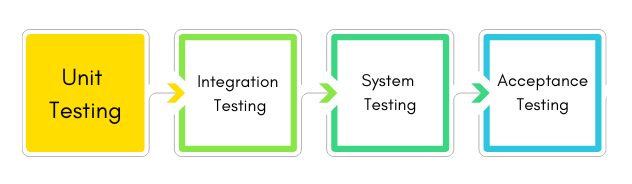
How to Perform Unit Testing
Developers use Unit Test to test a specific function in a software application by writing a code section. Developers can also isolate this function to test it more thoroughly, revealing unnecessary dependencies between the test function and other units that can be eliminated. Developers commonly use the UnitTest framework to create automated test cases for unit test.
There are two kinds of unit test:
- Manual Testing
- Automated Testing
Unit testing is frequently automated, but it can also be done manually. There is no preference in software engineering for one over the other, but automation is preferred. A step-by-step instructional document may be used in a manual approach to unit test.
Under the automated approach-
A developer writes code in the application to test the function. When the application is deployed, they will comment and eventually remove the test code.
A developer could also isolate the function to test it more thoroughly. This is a more comprehensive unit test practice that involves copying and pasting code to its testing environment rather than its natural environment. Isolating the code aids in discovering unneeded dependencies between the code under test and other units or data spaces in the product. These reliances can then be removed.
A coder will typically use a UnitTest Framework to create automated test cases. The developer uses an automation framework to code criteria into the test to ensure that the code is correct. The framework logs failing test cases while they are being executed. Many frameworks will also flag and report these failed test cases automatically. The framework may halt subsequent testing depending on the severity of a failure.
Unit test workflow is as follows:
1) Create Test Cases
2) Rework/Review
3) Baseline
4) Carry out test cases.
Unit Testing Techniques
Unit testing techniques are divided into three categories: Black box testing, white box testing, and grey box testing.
- Black box testing involves testing the user interface as well as input and output,
- White box testing involves testing the functional behaviour of the software application.
- Grey box testing involves executing test suites, test methods, test cases and performing risk analysis.
Code coverage techniques used in Unit Test are listed below:
- Statement Coverage
- Decision Coverage
- Branch Coverage
- Condition Coverage
- Finite State Machine Coverage
Read more: Different Types of Software Testing
Unit Test Example: Mock Objects
Mock objects are used in unit test to test sections of code that are not yet part of a complete application. Mock things stand in for missing program components.
For example, you could have a function that requires variables or objects that have not yet been created. Those will be accounted for in unit test as mock objects created solely for unit test on that section of code.
Unit Testing Tools
Various automated unit test software is available to help with unit test. We’ll give you a few examples below:
Junit is a free testing tool for the Java programming language. It includes assertions to help identify the test method—this tool tests data before inserting it into the code.
NUnit: NUnit is a popular unit-testing framework for all.net languages. It is an open-source tool that allows you to write scripts manually. It supports data-driven tests that can run concurrently.
JMockit is a free and open-source unit test tool. It’s a code coverage tool that includes line and path metrics. It enables API mocking with recording and verification syntax. Line coverage, Path coverage, and Data coverage are all provided by this tool.
EMMA: EMMA is a free, open-source toolkit for analyzing and reporting Java code. Emma accepts coverage types such as method, line, and basic block. Because it is Java-based, it does not require any external libraries and has access to the source code.
PHPUnit is a unit testing tool for PHP developers. It takes small sections of code known as units and tests them individually. The tool also enables developers to assert that a system behaves in a specific way using pre-defined assertion methods.
These are just a few of the unit test tools available. There are many more, particularly for C and Java, but regardless of your language, you will find a unit test tool that meets your needs.
Unit Testing and Test Driven Development (TDD)
Unit testing in TDD involves an extensive use of testing frameworks. To create automated unit tests, a unit test framework is used. TDD does not require unit testing frameworks, but they are necessary. Here are some of the benefits of TDD in the world of unit test:
- Tests are written before the code.
- Rely heavily on testing frameworks
- All classes in the applications are tested.
- Quick and easy integration is made possible.
Unit Testing Myth
Myth: It requires time, and I am always overscheduled. My code is rock solid! I do not need unit tests.
Myths, by their very nature, are false assumptions. These assumptions lead to a vicious cycle as follows –
The truth is that unit test accelerates development.
Programmers believe that Integration Testing will catch all errors and do not run unit tests. Once units are integrated, very simple mistakes that could have been found and fixed quickly in unit testing take a long time to trace and fix.
Advantage
Developers interested in learning what functionality a unit provides and how to use it can look at the unit tests to understand the unit API.
Unit testing enables the programmer to refactor code later while ensuring that the module continues to function correctly (i.e. Regression testing). The procedure is to write test cases for all functions and methods so that any changes that cause a bug can be quickly identified and fixed.
Because unit test is modular, we can test parts of the project while others are being completed.
Disadvantages
- It is unrealistic to expect unit test to catch every error in a program. Even in the most straightforward programs, it is impossible to evaluate all execution paths.
- Unit test, by definition, focuses on a single piece of code. As a result, it cannot detect integration or system-wide errors.
Unit test should be used in conjunction with other testing activities.
Best Practices
- Unit test cases should be self-contained. Unit test cases should not be affected by any requirements changes.
- Only test one code at a time.
- For your unit tests, use clear and consistent naming conventions.
- Before changing the implementation of any module, ensure that there is a corresponding unit Test Case for the module and that the module passes the tests.
- Bugs discovered during unit test must be fixed before moving on to the next phase of the SDLC.
- Adopt a “test as code” strategy. The more code you write without testing, the more paths you’ll have to go through to check for errors.
Summary
Unit Testing is a type of software testing in which individual software units or components are tested.
As you can see, unit test can be pretty complex. Depending on the application being tested and the testing strategies, tools, and philosophies employed, it can be complex or relatively simple. Unit testing is always required at some level. That is a foregone conclusion.